Lifecycle Hooks in Lightning Web Components
- Saurabh Singh
- May 2, 2019
- 3 min read
Updated: Apr 17, 2020
In Aura framework init(), render(), rerender(), afterRender(), unrender() methods were used to handle the lifecycle of components. Similarly, Lightning web components have callback method, that are used to handle lifecycle of the components. These are called lifecycle hooks. You can override these hooks to modify the behavior.
1. constructor() -Constructor fires when a component instance is created, it is similar to init() method of aura component.
Do not assign any property in Constructor because they are not ready at this point.The flow of Constructor is flows from parent to child (init flows from child to parent), so do not try to access child element. It means if there are child components in LWC, then first parent constructor will be called, followed by child constructor.
import { LightningElement } from 'lwc';
export default class App extends LightningElement {
constructor() {
super();
console.log('In Constructor');
}
2. connectedCallback() -
This method fires when component inserted into DOM, and it can fire more than one time.This hook fires from parent to child. You can’t access child elements in the component body because they don’t exist yet.Parent element can be modified in this hook
import { LightningElement, track, api } from 'lwc';
export default class App extends LightningElement {
lst=[];
constructor() {
super();
console.log('In Constructor');
}
connectedCallback() {
this.lst.push('Hello LWC');
console.log('ConnectedCallback');
console.log(this.lst);
}
}

3. disconnectedCallback() -
It fires when a component removed from DOM.This hook flows from parent to child.This hook is similar to unrender() method of aura component.
import { LightningElement, track, api } from 'lwc';
export default class App extends LightningElement {
lst=[];
constructor() {
super();
console.log('In Constructor');
}
connectedCallback() {
this.lst.push('Hello LWC');
console.log('ConnectedCallback');
console.log(this.lst);
}
disconnectedCallback() {
this.lst=[]
console.log('disconnectedCallback');
}
}
When this component removed form DOM, it will fire disconnectedCallback and clear array.
4. render() -
This method is used for conditional renderingThis function gets invoked after connectedCallback() and must return a valid HTML template.
<!-- temp1.html -->
<template><h1>Hello This is Template 1 !</h1><lightning-buttononclick={changeTemp}label="GoTo Template 2" name="btn1"
></lightning-button>
</template>
<!-- temp2.html -->
<template><h1>Hello this is Template 2</h1><lightning-buttononclick={changeTemp}label="GoTo Template 1" name="btn2"
></lightning-button>
</template>
app.html can be empty
<!-- app.html -->
<template>
</template>
// app.js
import { LightningElement, track, api } from 'lwc';
import page1 from './temp1.html';
import page2 from './temp2.html';
export default class App extends LightningElement {
lst=[];
@api page = 'temp1';
constructor() {
super();
console.log('In Constructor');
}
connectedCallback() {
this.lst.push('Hello LWC');
console.log('ConnectedCallback');
console.log(this.lst);
}
disconnectedCallback() {
this.lst=[];
console.log('disconnectedCallback');
}
changeTemp() {
if(this.page=='temp1')
this.page='temp2';
elsethis.page='temp1';
}
render() {
if(this.page=='temp1')
return page1;
elsereturn page2;
}
}
Output :-
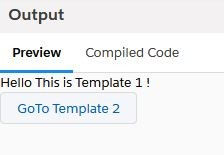
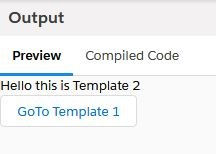
5. renderedCallback() -
This method is called after render() method. For every render of the component, this method will be fired.This hook flows from child to parent.This hook is called after every render of the component, so you need to be careful, if you want to perform some operation is specific conditions. It should be guarded with conditions that do not trigger an infinite rendering loop.
import { LightningElement, track, api } from 'lwc';
import page1 from './temp1.html';
import page2 from './temp2.html';
export default class App extends LightningElement {
lst=[];
@api page = 'temp1';
constructor() {
super();
console.log('In Constructor');
}
connectedCallback() {
this.lst.push('Hello LWC');
console.log('ConnectedCallback');
console.log(this.lst);
}
disconnectedCallback() {
this.lst=[];
console.log('disconnectedCallback');
}
changeTemp() {
if(this.page=='temp1')
this.page='temp2';
elsethis.page='temp1';
}
render() {
if(this.page=='temp1')
return page1;
elsereturn page2;
}
renderedCallback(){
console.log("renderedCallback");
if(this.page == 'temp2')
{
console.log(this.page);
var btn=this.template.querySelectorAll("lightning-button");
btn.forEach(function(element){
console.log(btn.name);
if(element.name=='btn2')
{
element.variant="success";
}
},this);
}
}
}
In this code renderedCallback method, check if it is second page, then change the button variant to success.
Output :-
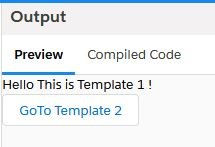

6. errorCallback(error, stack) -
Called when a descendant component throws an error in one of its lifecycle hooks.The error argument is a JavaScript native error object, and the stack argument is a string. The method works like a JavaScript catch{} block for components that throw errors in their lifecycle hooks. This hook capture only from child component, and not from itself.
errorCallback(error, stack) {
alert(error);
}
when any descendant component throw any error, this method will capture and show an alert. You can access LWC documentation to know more about lifecycle hooks.
Moreover you can check lifecycle flow diagram to understand, what parent to child, and child to parent flow means.
Commenti